您现在的位置是:java学习笔记 >
java学习笔记
java时间戳转换日期格式精确到毫秒的代码实现
本 文 目 录
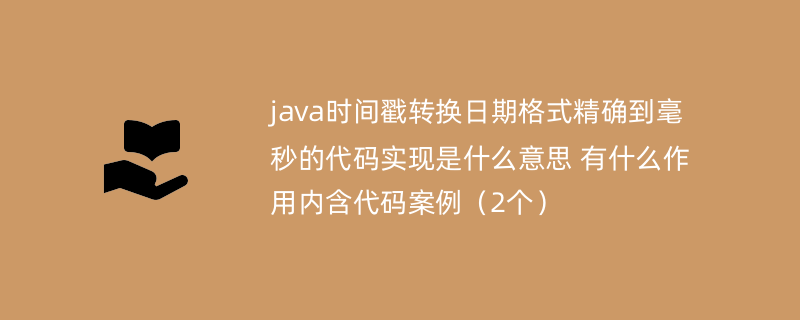
一、Java时间戳转换日期格式精确到毫秒代码案例及分析
案例一:使用Java 8的LocalDateTime和Instant类
Java 8引入了LocalDateTime
和Instant
类,这两个类可以帮助我们轻松地将时间戳转换为日期格式,并且精确到毫秒。
代码实现
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class TimestampToDateExample {
public static void main(String[] args) {
long timestamp = System.currentTimeMillis(); // 获取当前时间戳
Instant instant = Instant.ofEpochMilli(timestamp); // 将时间戳转换为Instant对象
LocalDateTime dateTime = LocalDateTime.ofInstant(instant, java.time.ZoneId.systemDefault()); // 将Instant对象转换为LocalDateTime对象
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss.SSS"); // 设置日期格式
String dateString = dateTime.format(formatter); // 将LocalDateTime对象格式化为字符串,精确到毫秒
System.out.println(dateString);
}
}
分析:
System.currentTimeMillis()
方法用于获取当前的时间戳,返回的是一个long类型的值,单位是毫秒。Instant.ofEpochMilli(timestamp)
方法将时间戳转换为Instant
对象,Instant
表示一个精确到纳秒的时间点。LocalDateTime.ofInstant(instant, java.time.ZoneId.systemDefault())
方法将Instant
对象转换为LocalDateTime
对象,默认的时区是系统默认的时区。DateTimeFormatter
用于指定日期和时间的格式,通过format()
方法可以将LocalDateTime
对象按照指定的格式转换成字符串。这里我们使用的是"yyyy-MM-dd HH:mm:ss.SSS"这个格式,精确到毫秒。
案例二:使用Java 8的ZonedDateTime和Duration类
对于需要处理时区的情况,我们可以使用ZonedDateTime
和Duration
类。
代码实现
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.time.Duration;
import java.util.concurrent.TimeUnit;
public class TimestampToDateWithTimeZoneExample {
public static void main(String[] args) {
long timestamp = System.currentTimeMillis(); // 获取当前时间戳,这里的时间戳不考虑时区问题
ZonedDateTime zonedDateTime = ZonedDateTime.ofInstant(Instant.ofEpochMilli(timestamp), ZoneId.systemDefault()); // 将时间戳转换为ZonedDateTime对象,考虑到时区问题
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss.SSS"); // 设置日期格式,同样精确到毫秒
String dateString = zonedDateTime.format(formatter); // 将ZonedDateTime对象格式化为字符串,精确到毫秒,考虑到了时区问题
System.out.println(dateString);
}
}
分析:
ZoneId.systemDefault()
方法用于获取系统默认的时区。这里注意与案例一的区别,我们需要考虑时区的问题。如果不考虑时区的问题,可以使用案例一中的方法。ZonedDateTime
对象表示一个特定的时间和地点,包括时间和地点的具体信息。这里我们使用的是系统默认的时区。Duration
类用于表示时间的长度,可以用来计算两个时间点之间的差值。这里我们用来表示毫秒级别的差值。format()
方法同样可以将ZonedDateTime
对象按照指定的格式转换成字符串,这里我们使用的是"yyyy-MM-dd HH:mm:ss.SSS"这个格式,精确到毫秒。同时考虑到了时区的问题。
二、相关知识及使用技巧
相关知识:Java 8中的日期和时间类库(java.time包)
Java 8引入了全新的日期和时间类库,包括LocalDate
、LocalTime
、LocalDateTime
、ZonedDateTime
、Instant
等类,这些类可以帮助我们更方便地处理日期和时间相关的操作。其中,LocalDateTime
和ZonedDateTime
类可以帮助我们处理精确到毫秒。
- 上一篇
java将某个日期转为时间戳代码
## 一、Java将某个日期转为时间戳代码Java提供了DateTimeFormatter类来处理日期和时间。可以使用该类将日期对象转换为时间戳。以下是一个简单的示例代码:```javaimport java.time.LocalDate;import java.time.ZoneId;import java.time.ZonedDateTime;import java.time.Instant;
- 下一篇
java比较两个日期是否相等的代码
Java提供了多种方法来比较两个日期是否相等,但哪种方法最适合你的特定需求呢?在这篇文章中,我们将深入探讨Java中比较两个日期是否相等的代码,并提供两个代码案例,以及相关的使用技巧。## 一、Java比较两个日期是否相等的代码------------在Java中,可以使用以下方法来比较两个日期是否相等:### 方法一:使用`Date`类的`equals()`方法```javaimport jav