架构师问答
Java List集合到数组的转换
本 文 目 录
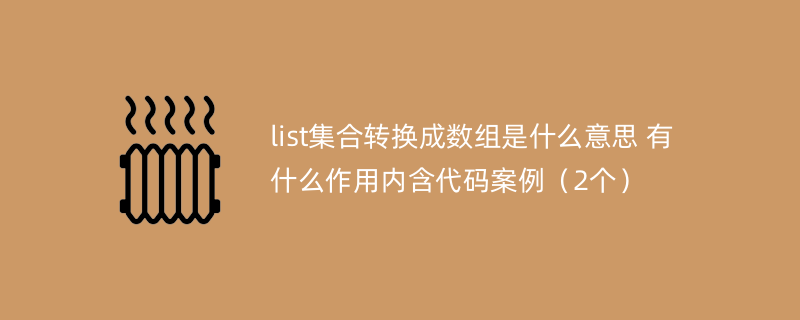
Java集合框架是一个非常强大的工具,提供了各种不同的集合类型,包括列表(List)、集合(Set)、映射(Map)
等。在Java中,一个常见的任务是将List集合转换成数组。这在许多情况下是非常有用的,例如在进行算法实现、数据处理或参数传递时。在本篇文章中,我们将详细介绍如何将Java List集合转换为数组,并分享两个实用的代码案例。
一、List集合转换为数组的基本知识
List集合是Java集合框架中的一个接口,它表示一个有序的集合,其中每个元素都有一个特定的索引。Java中提供了一些实现List接口的类,如ArrayList和LinkedList。而数组则是一种简单的数据结构,可以存储多个相同类型的数据,通过索引访问元素。
要将List集合转换为数组,可以使用Java中的toArray()方法。该方法将List集合转换为一个新的数组。请注意,这个方法并不会改变原始的List集合。此外,你也可以使用Arrays类中的asList()方法将List集合转换为数组。
二、List集合到数组的转换代码案例及分析
案例一:使用toArray()方法
假设我们有一个名为students的List集合,它包含一些学生的姓名和年龄。我们希望将这个List转换为数组。以下是一个示例代码:
import java.util.*;
public class Main {
public static void main(String[] args) {
// 创建一个List集合
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 20));
students.add(new Student("Bob", 22));
students.add(new Student("Charlie", 25));
// 将List转换为数组
Student[] studentsArray = students.toArray(new Student[0]);
// 打印数组内容
for (Student student : studentsArray) {
System.out.println(student.getName() + " " + student.getAge());
}
}
}
class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
在这个例子中,我们使用了toArray()
方法将List<Student>
转换为Student[]
数组。需要注意的是,toArray()方法的参数是一个空的Student数组,它的长度必须大于等于List中元素的数量,否则会抛出ArrayIndexOutOfBoundsException
异常。此外,toArray()方法返回的是Object[]数组,因此我们需要显式地指定要转换的类型(在这个例子中是Student[])。最后,我们使用for-each循环遍历并打印出数组中的所有元素。
案例二:使用Arrays.asList()方法
另一种将List转换为数组的方法是使用Arrays类的asList()方法。该方法返回一个固定大小的列表(Arraylist),其中包含原始List的所有元素。以下是一个示例代码:
import java.util.*;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// 创建一个List集合
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// 将List转换为数组(固定大小的列表)
String[] namesArray = Arrays.copyOf(names.toArray(new String[0]), names.size());
// 打印数组内容
for (String name : namesArray) {
System.out.println(name);
}
}
}
在这个例子中,我们使用了Arrays.asList()
方法将List<String>
转换为String[]数组
。需要注意的是,这种方法返回的是固定大小的列表(Arraylist),而不是动态数组。因此,在使用前需要确保列表的大小不会超过数组的大小。另外,我们使用Arrays.copyOf()
方法复制列表中的所有元素到一个新的数组中。这个方法会创建一个新的数组,并将列表中的所有元素复制到新数组中。最后,我们使用for-each
循环遍历并打印出数组中的所有元素。
- 上一篇
JavaList集合转换成JSON字符串详解
标题:JavaList集合转换成JSON字符串详解在Java中,将JavaList集合转换为JSON字符串是一种常见的操作,这对于数据交换和网络通信非常有用。在本文中,我们将介绍如何使用Java中的一些库将JavaList集合转换为JSON字符串,并讨论相关的知识和使用技巧。## 一、关于java中json与list集合的相关知识1. Java中的JSON库:在Java中,有许多库可用于将Java
- 下一篇
Java将List集合转化为字符串详解
在Java中,我们经常需要将各种数据结构,如数组、集合等,转化为字符串以便于存储、传输或打印。对于List集合,这种需求尤为常见。Java提供了多种方法可以将List集合转化为字符串,其中最常用的是使用`toString()`方法。然而,这种方法可能无法满足所有需求,因此我们需要掌握一些额外的技巧和方法。## 一、List集合转化为字符串的基本方法---------------------###